In this article, I will introduce two techniques to handle multiple requests in Angular by using mergeMap and forkJoin.
Contents:
- Problem
subscribe
mergeMap
forkJoin
- Combine
mergeMap
andforkJoin
- Performance comparison of
subscribe
vsmergeMap
andforkJoin
Problem
In the real world, we frequently call more than one API in our web applications. When you enter a page, you often make multiple requests to retrieve all the required data, and the results of some API requests are required for subsequent calls.
When we make multiple requests, it’s important to handle them effectively to maintain fast performance for your users while also writing good code.
- Call the API to authenticate and retrieve user information
- Based on the user information, we call one API to get all posts created by the user.
- Based on the user information, we call one API to get all the albums created by the user.
subscribe
is a common way to handle requests in Angular, but there are more effective methods. We will first solve our problem using subscribe
and then improve on it using mergeMap
and forkJoin
Subscribe
Using this technique is quite simple. First, we call one API to get user info, and then we call the other two APIs. We do this in a nested subscription so we can use the results from the first API call.
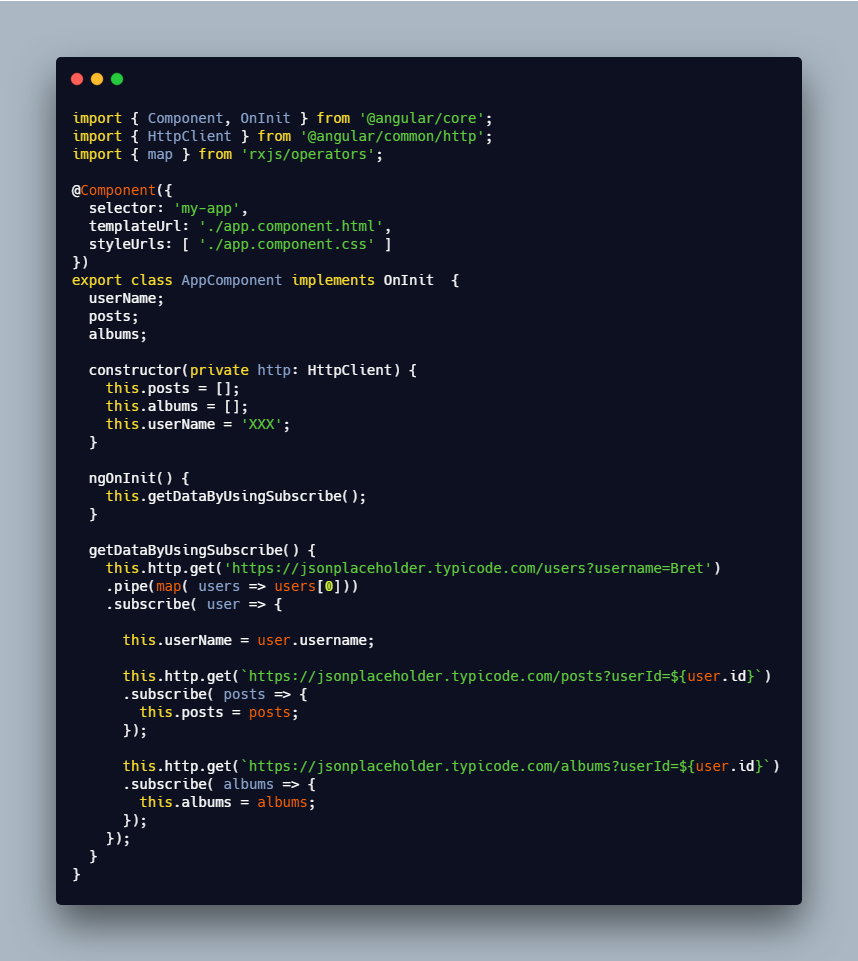
This technique is fine for 2 or 3 requests, but it is hard to read for more requests as your app grows. We would be required to create a lot of nested subscription. That is why we will use RxJS to handle multiple requests.
MergeMap
This operator is best used when you wish to flatten an inner observable but want to manually control the number of inner subscriptions.
So when do we apply
mergeMap
?
When we need data from the first API request to make requests to the second API.

Look at the source code above, we can see that second API needs the user ID from the first API to get data.
Note:
flatMap
is an alias formergeMap
.mergeMap
maintains multiple active inner subscriptions at once, so it’s possible to create a memory leak through long-lived inner subscriptions.
ForkJoin
This operator is best used when you have a group of observables and only care about the final emitted value of each. It means that
forkJoin
allows us to group multiple observables and execute them in parallel, then return only one observable.
When do we apply
forkJoin
?
We use it when API requests are independent. It means that they do not depend on each other to complete and can execute in parallel.

Combine mergeMap and forkJoin
In the real world, there are multiple API requests that depend on the result of another request. So let’s see how can we handle that case by using
mergeMap
and forkJoin
.
Here is a sample to solve our problem:

By using these functions, we avoided nested subscriptions and can split code into many small methods.
You have to replace
userId
inside mergeMap
by user
that return from map
above.
No comments:
Post a Comment